Last year I posted an article which showed how to store the files which are uploaded using UXFileUpload (ClientUI control for Silverlight and WPF) in database. In this article, I will show a similar scenario which can be done using WebFileUploader, a member of Intersoft Solutions ASP.NET controls.
WebFileUploader is an easy to use; high performance and advanced file upload component which allows you to upload multiple files without page refresh. WebFileUploader uses 100% HTML technology for all its features, and fully supports all modern browsers including Firefox, Safari, Chrome and Opera. Using 100% HTML technology means that WebFileUploader doesn’t require Flash plug-in to support multiple files uploading and many other features.
This scenario, to store the uploaded files in database, can be divided to two parts. The first part is to configure WebFileUploader for IIS application; and the other part, using server-side code to process uploaded files and store them into database.
To configure WebFileUploader for IIS application
Some configuration is needed in the web.config to run WebFileUploader in IIS. The list below shows how to configure WebFileUploader for IIS 7 application.
- By default, ASP.NET application restricts maximum request length to 4 MB. To enable our application to accept larger files, please configure the maxRequestLength in web.config to higher value. The maxRequestLength value is measured in kilobytes. Here is a snippet to increase your file size to 100MB.
<configuration>
<system.web>
<httpRuntime maxRequestLength="102400" />
</system.web>
</configuration>
- Add WebFileUploader handler to <handlers> section under <system.webServer>. Here is the snippet.
<add name="WebFileUploaderHttpHandler" verb="GET"
path="WebFileUploaderHttpHandler.axd"
type="ISNet.WebUI.WebTextEditor.WebFileUploaderHttpHandler, ISNet.WebUI.WebTextEditor"
preCondition="integratedMode" />
- Add WebFileUploader module to <modules> section under <system.webServer> in our project web.config. Here is the snippet.
<add name="WebFileUploaderHttpModule" preCondition="managedHandler"
type="ISNet.WebUI.WebTextEditor.WebFileUploaderHttpModule, ISNet.WebUI.WebTextEditor" />
- Set maxAllowedContentLength to a higher value, which is measured in bytes, to 100MB in web.config. This attribute specifies the maximum length of content in a request. The default value is 30000000, which is approximately 28.6 MB.
<system.webServer>
<security>
<requestFiltering>
<requestLimits maxAllowedContentLength="104857600" />
</requestFiltering>
</security>
</system.webServer>
- Unlock the mode override in applicationHost.config. Open IIS 7 application configuration file, the default location is in C:\windows\system32\inetsrv\config, and change the overrideModeDefault to Allow.
<section name="requestFiltering" overrideModeDefault="Allow" />
Save the changes and then try to upload a file. If WebFileUploader has been successfully uploaded the file to the designated folder, it means that we are on half way to achieve the goal and ready to proceed to the next step.
Using server-side code to process uploaded files and store them into database
A database, called Files.mdf, is added into the App_Data folder of the web project. This database has Files table which consists of following fields.
Column Name |
Data Type |
Allow Nulls |
Id |
uniqueidentifier |
False |
FileData |
varbinary(MAX) |
True |
OriginalName |
nvarchar(50) |
False |
DateCreated |
datetime |
False |
A Stored Procedure, sprocFilesInsertSingleItem, will be used to insert a single item of file into Files.mdf database.
INSERT INTO Files
(
Id,
FileUrl,
FileData,
OriginalName
)
VALUES
(
@id,
@FileUrl,
@FileData,
@originalName
)
Next, we are going to use the OnAfterUpload server-side event of WebFileUploader. It is the server-side event which fired when a file upload is succeeded. Generally, there are three processes to be performed on this event.
- Read and manipulate the uploaded file using FileStream class.
- Invoke and execute sprocFilesInsertSingleItem stored procedure to store the uploaded file on Files.mdf database.
- Delete the specified file from the UploadPath folder.
protected void WebFileUploader1_AfterUpload(object sender, ISNet.WebUI.WebTextEditor.WebFileUploaderFileEventArgs e)
{
byte[] fileData = ReadFile(e.WebFileUploadInfo.Path + "\\" + e.WebFileUploadInfo.FileName);
string originalName = e.WebFileUploadInfo.FileName;
using (SqlConnection mySqlConnection = new SqlConnection(@"Data Source=.\SQLEXPRESS;AttachDbFilename=|DataDirectory|\Files.mdf;Integrated Security=True;Connect Timeout=30;User Instance=True"))
{
// Set up the Command object
SqlCommand myCommand = new SqlCommand("sprocFilesInsertSingleItem", mySqlConnection);
myCommand.CommandType = CommandType.StoredProcedure;
// Set up the ID parameter
SqlParameter prmId = new SqlParameter("@id", SqlDbType.UniqueIdentifier);
Guid id = Guid.NewGuid();
prmId.Value = id;
myCommand.Parameters.Add(prmId);
// Set up the FileUrl parameter
SqlParameter prmFileUrl = new SqlParameter("@fileUrl", SqlDbType.NVarChar, 255);
prmFileUrl.Value = DBNull.Value;
myCommand.Parameters.Add(prmFileUrl);
// Set up the FileData parameter
SqlParameter prmFileData = new SqlParameter("@fileData ", SqlDbType.VarBinary);
prmFileData.Value = fileData;
prmFileData.Size = fileData.Length;
myCommand.Parameters.Add(prmFileData);
// Set up the OriginalName parameter
SqlParameter prmOriginalName = new SqlParameter("@originalName", SqlDbType.NVarChar, 50);
prmOriginalName.Value = e.WebFileUploadInfo.FileName;
myCommand.Parameters.Add(prmOriginalName);
// Execute the command, and clean up.
mySqlConnection.Open();
bool result = myCommand.ExecuteNonQuery() > 0;
mySqlConnection.Close();
}
File.Delete(e.WebFileUploadInfo.Path + "\\" + e.WebFileUploadInfo.FileName);
}
private static byte[] ReadFile(string filePath)
{
byte[] buffer;
FileStream fileStream = new FileStream(filePath, FileMode.Open, FileAccess.Read);
try
{
int length = (int)fileStream.Length; // get file length
buffer = new byte[length]; // create buffer
int count; // actual number of bytes read
int sum = 0; // total number of bytes read
// read until Read method returns 0 (end of the stream has been reached)
while ((count = fileStream.Read(buffer, sum, length - sum)) > 0)
{
sum += count; // sum is a buffer offset for next reading
}
}
finally
{
fileStream.Close();
}
return buffer;
}
That’s it! Now the files uploaded by WebFileUploader control will be stored in database. It’s pretty easy and straightforward, don’t you think so?
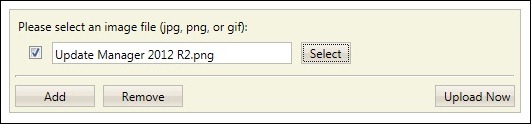
Click here to download the sample and feel free to drop me a line in the comment box if you find this post useful.